Integrating with Hesabe Payment Gateway API End-point
This guide will help you integrate your application or platform with Hesabe’s Payment Gateway API.
Integration Types
Direct Integration
In this integration method, the merchant provides an option to select the Payment Method (Knet or MPGS) in the app itself. Based on the customer’s selection, the request param paymentType should be sent accordingly as described in the section Posting Payment Data.
Indirect Integration
In this integration method, the merchant does not provide an option to select the Payment Method (Knet or MPGS) in the app. Customers would be able to select their choice of Payment Method from the Hesabe Payment Gateway Landing page. The request param paymentType should be sent accordingly as described in the section Posting Payment Data.
Based on the selection of Payment Method here, the Customer will be redirected to the respective Payment page and Payment Type will be recorded by Hesabe.
The Hesabe Payment Gateway Page would look similar to as shown below:
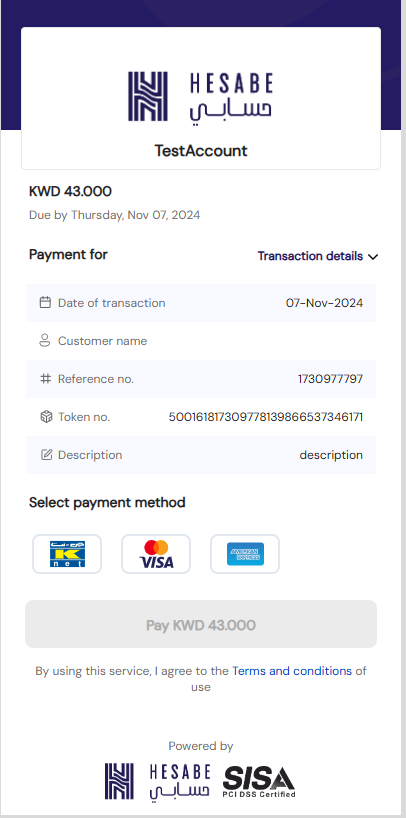
Posting Payment Data
Payment data containing the following fields in encrypted form need to be submitted to ‘Checkout’ Endpoint (https://sandbox.hesabe.com/checkout on Test and https://api.hesabe.com/checkout on Production) for initiating the payment request.
Environment | URL |
---|---|
Test | https://sandbox.hesabe.com/checkout |
Production | https://api.hesabe.com/checkout |
Request Parameters
Field | Type | Description |
---|---|---|
amount | Numeric | Amount in currency |
currency | String | ISO currency code |
responseUrl | String | Redirect URL on success |
failureUrl | String | Redirect URL on failure |
merchantCode | Numeric | Assigned by Hesabe |
paymentType | Numeric | 0 = Indirect, 1 = KNET, 2 = Visa/MasterCard, 7 = Amex, 9 = Apple Pay |
version | String | API version (e.g., 2.0 ) |
orderReferenceNumber | String | Your reference/order ID |
variable1-5 | String | Optional key-value pairs (Label__Value ) |
variable2 | String | Optional key-value pairs (Label__Value ) |
variable2 | String | Optional key-value pairs (Label__Value ) |
variable3 | String | Optional key-value pairs (Label__Value ) |
variable4 | String | Optional key-value pairs (Label__Value ) |
variable5 | String | Optional key-value pairs (Label__Value ) |
name* | String | Customer name |
mobile_number* | Numeric (8) | Customer mobile (no country code) |
email* | String | Customer email |
saveCard# | Boolean | Save card for tokenization |
cardId# | String | Tokenized card ID |
authorize~ | Boolean | Authorization-only transaction |
webhookUrl | String | Your endpoint for receiving payment status |
* These parameters are only used for KFast.
If you want to enable KFast, please contact your Account Manager or email support@hesabe.com.
# These parameters are only used for Tokenization using Cybersource & MPGS.
To enable this, please contact your Account Manager or email support@hesabe.com.
~ These parameters are only used for Authorization-only transactions using MPGS.
To enable this feature, contact your Account Manager or email support@hesabe.com.
Params - variable1, variable2, variable3, variable4, and variable5 can be used to pass arbitrary values (string only) in the form of key value pairs in the format "key__value". For example, to pass Customer Name in variable1, you can pass "Customer Name__John Doe" as value of variable1. These values would then be visible in the transaction details window of Merchant Panel and also be displayed in the email notifications.
The data is posted/passed to the HesabeRequestHandler that will concatenate and encrypt the data before invoking the Hesabe Checkout API endpoint.
1. Purchase / Sales
Steps to Integrate Hesabe Payment Gateway:
-
Sign Up with Hesabe and get a Merchant Account created and approved.
-
Login to the
Merchant Panel
using the credentials provided after registration. -
Download the Hesabe Payment Integration Kit (currently available for PHP; other platforms will be added soon).
-
Copy the
Encryption Key
from the Account section. -
The
Access Code
&IV Key
will be shared by Hesabe. -
Configure Hesabe security keys in your application.
-
Create a payment form and deploy request/response handlers with the guidance of the integration kit.
-
Create return pages for handling payment notifications on success or cancellation.
Download Hesabe Payment Gateway Integration Kit
The Hesabe payment gateway integration kit is a client library provided by Hesabe.
After signing in with the approved Merchant account, you can download the Integration Kit. From the Merchant Panel Dashboard, navigate through Resources > Download Integration Kit > Download PHP to get the integration kit for PHP.
The integration kit contains request and response payment handler files. It also includes HesabeCrypto.php
, which provides functions to encrypt and decrypt merchant data using AES-256-CBC.
Access Hesabe Merchant ID, Access Code, and Encryption Keys
To implement Hesabe payment gateway integration, the following credentials are required:
- Merchant ID
- Access Code
- Secret Key
- IV Key
These can be obtained after activating your Hesabe Merchant account.
After logging into the Hesabe Merchant Panel using your merchant account credentials:
-
Navigate to the Account section.
-
Under Security Keys, you will find the Merchant ID, Access Code, and Secret Key. The IV Key will be shared along with the credentials to access the Merchant Dashboard after account activation.
Integrating with Hesabe Payment Gateway API End-point
Posting Payment Data
Payment data containing the specified fields in encrypted form needs to be submitted during checkout to initiate the payment request.
Request Parameters
Name | Description | Condition | Type/Length |
---|---|---|---|
amount | Amount must be greater than zero | Required | Numeric |
responseUrl | URL to which the user will be redirected after payment completion | Required | Alphanumeric (200) |
failureUrl | URL to which the user will be redirected if the payment is declined | Required | Alphanumeric (200) |
merchantCode | Unique identifier generated by Hesabe for each activated merchant | Required | Numeric |
access_code | Access code used to revalidate a Merchant (only for Indirect Integration) | Required | Alphanumeric (32) |
paymentType | Payment Type: 0 - Indirect, 1 - Knet, 2 - MPGS | Required | Numeric |
version | Version of the API (e.g., 2.0) | Required | Alphanumeric (10) |
variable1 | Custom user parameters included in the response | Optional | Alphanumeric (100) |
variable2 | Custom user parameters included in the response | Optional | Alphanumeric (100) |
variable3 | Custom user parameters included in the response | Optional | Alphanumeric (100) |
variable4 | Custom user parameters included in the response | Optional | Alphanumeric (100) |
variable5 | Custom user parameters included in the response | Optional | Alphanumeric (100) |
The data is posted to the HesabeRequestHandler
, which concatenates and encrypts the data before invoking the Hesabe Payment API endpoint.
Customer Details
To add customer details to the transaction, include the following parameters in the request:
name
: Customer's name (Alphanumeric, max 100 characters)mobile_number
: Customer's mobile number without country code (Numeric, 8 digits)email
: Customer's email address (Alphanumeric, max 100 characters)
Webhook URL Response
To receive transaction responses, add the following parameter:
webhookUrl
: Your page URL for receiving transaction updates. For more details, refer to the Webhook Integration.
2. Authorize / Capture
Overview
The Authorize/Capture payment flow is a two-step process used in payment processing. It allows a merchant to authorize a transaction first, ensuring that the funds are available in the customer’s account, and then capture the funds at a later time when the service or product is ready to be delivered.
Note: Authorize and Capture features are only available with the MPGS payment gateway service.
Authorize Transaction Request
To authorize a transaction, include the following parameter in the checkout request:
authorize
: Boolean flag to indicate authorization.
Capture Transaction Request
To capture the authorized transaction, send a POST request with the following parameters:
merchantCode
: Unique identifier generated by Hesabe for each activated merchant.paymentToken
: Payment Token returned from the authorized transaction.amount
: Transaction amount equal to or less than the authorized transaction amount.
Sample PHP Code:
$baseUrl = 'http://sandbox.hesabe.com/api/';
$captureApiUrl = $baseUrl . 'capture';
$requestData = [
'merchantCode' => 842217,
'amount' => 10,
'paymentToken' => '84221717175011419773484853173'
];
$encryptedData = HesabeCrypto::encrypt($requestData, $encryptionKey, $ivKey);
$checkoutRequestData = new Request([
'data' => $encryptedData
]);
$checkoutRequest = Request::create($captureApiUrl, 'POST', $checkoutRequestData->all());
$checkoutRequest->headers->set('accessCode', $accessCode);
Response Sample
{
"status": true,
"message": "Your refund request is waiting for approval",
"data": {
"token": "521042117249344539468767555844",
"amount": "1.000",
"order_reference_number": "1724934434",
"transaction_status": "SUCCESSFUL",
"refund_status": "PENDING",
"payment_type": "KNET",
"service_type": "Payment Gateway",
"transaction_datetime": "2024-08-29 15:27:38",
"refund_requested_at": "2024-09-04 15:04:44"
}
}
Cancel Authorized Transaction
To cancel the Authorized transaction for further Capturing the Amount.
$baseUrl = http://sandbox.hesebe.com/api/
$CancelApiUrl = {{$baseUrl}}/cancel/{paymentToken}
$checkoutRequest = Request::create($CaptureApiUrl, 'DELETE');
$checkoutRequest->headers->set('accessCode', $accessCode);
3. Add Card / Merchant Initiated Transaction
Overview
The Add Card and Merchant Initiated Transaction (MIT) processes are essential components in payment systems, particularly for businesses that require recurring billing, subscriptions, or the ability to charge customers without their direct input at the time of each transaction.
Add Card
To allow users to add their card in your app or website, create a checkout request with the following parameters and set the amount to zero.
Note: This feature will only work with the Cybersource Recurring Payment Gateway.
Parameter | Value |
---|---|
paymentType | 15 |
subscription | 1 |
amount | 0 |
Merchant Initiated Transaction
To initiate a merchant-triggered transaction using stored card credentials, create a checkout request using these parameters:
Parameter | Description |
---|---|
merchantCode | Merchant Code is a unique identifier generated by Hesabe for each activated merchant. |
paymentToken | Payment Token returned from Add Card Transaction |
Amount | Any transaction amount |
orderReferenceNumber | Custom user parameter which will be included in the response when it returns |
$baseUrl = http://sandbox.hesebe.com/api/
$CaptureApiUrl = {{$baseUrl}}/subscription/capture
$requestData = [
"merchantCode" => 842217
"amount" => 10,
"paymentToken"=>84221717175011419773484853173,
"orderReferenceNumber"=>12365478989
];
$encryptedData = HesabeCrypto::encrypt($requestData, $encryptionKey, $ivKey)
$checkoutRequestData = new Request([
'data' => $encryptedData
]);
$checkoutRequest = Request::create($CaptureApiUrl, 'POST', $checkoutRequestData->all());
$checkoutRequest->headers->set('accessCode', $accessCode);
Cancel Card
To cancel the Saved Card for further Transactions.
$baseUrl = http://sandbox.hesebe.com/api/
$CancelApiUrl = {{$baseUrl}}/subscription/cancel/{paymentToken}
$checkoutRequest = Request::create($CaptureApiUrl, 'DELETE');
$checkoutRequest->headers->set('accessCode', $accessCode);
4. Refund
Overview
A refund is a transaction process where a merchant returns funds to a customer after a payment has been processed.
Create a Refund Request
To create a refund request for a transaction, pass the following parameters:
Parameter | Description |
---|---|
merchantCode | Merchant Code is a unique identifier generated by Hesabe for each activated merchant. |
token | Transaction Token of the original transaction. |
refundAmount | Amount to be refunded. |
refundMethod | 1 for Full Refund, 2 for Partial Refund. |
$baseUrl = 'http://sandbox.hesabe.com/api/';
$refundRequestApiUrl = $baseUrl . 'transaction-refund';
$data = [
'merchantCode' => 'your_merchant_code',
'token' => '521042117249344539468767555844',
'refundAmount' => 1,
'refundMethod' => 2,
];
$checkoutRequest = Request::create($refundRequestApiUrl, 'POST', $data);
$checkoutRequest->headers->set('accessCode', $accessCode);
Response Sample
{
"status": true,
"message": "Your refund request is waiting for approval",
"data": {
"token": "521042117249344539468767555844",
"amount": "1.000",
"order_reference_number": "1724934434",
"transaction_status": "SUCCESSFUL",
"refund_status": "PENDING",
"payment_type": "KNET",
"service_type": "Payment Gateway",
"transaction_datetime": "2024-08-29 15:27:38",
"refund_requested_at": "2024-09-04 15:04:44"
}
}
5. Re-query
StartFragmentIf you wish to enquire about a transaction status, after completion, you can do so by using the below information provided to you:
Request Parameters
Field | Type | Description |
---|---|---|
data | Alphanumeric | Data can be either Payment Transaction token or Order Reference Number (used in the request) to be passed in the URL. NOTE: If you are using Order Reference Number, you are required to pass isOrderReference=1 in the body of the request. |
accessCode | String | Access Code provided by Hesabe to be passed in the Headers |
$baseUrl = https://sandbox.hesabe.com
$transactionApiUrl = {{$baseUrl}}/api/transaction/{{$data}}
$transactionRequestData = new Request();
$transactionRequest = Request::create($transactionApiUrl, 'POST', $transactionRequestData->all());
$transactionRequest->headers->set('accessCode', $accessCode);
$transactionRequest->headers->set('Accept', 'application/json');
$transactionResponse = Route::dispatch($transactionRequest);
$transactionResponseContent = $transactionResponse->content();
Response Sample
{
"status": true,
"message": "Transaction found",
"data": {
"token": "521042117249344539468767555844",
"amount": "45.000",
"reference_number": "1724934434",
"status": "SUCCESSFUL",
"TransactionID": "424210001296274",
"Id": 129179,
"PaymentID": "100424210000015649",
"Terminal": "144301",
"TrackID": "25119",
"payment_type": "KNET",
"service_type": "Payment Gateway",
"customerName": "User Name",
"customerEmail": "user@gmail.com",
"customerMobile": "98726012",
"customerCardType": null,
"customerCard": null,
"datetime": "2024-08-29 15:27:38"
},
"results": [
{
"token": "521042117249344539468767555844",
"amount": "45.000",
"reference_number": "1724934434",
"status": "SUCCESSFUL",
"TransactionID": "424210001296274",
"Id": 129179,
"PaymentID": "100424210000015649",
"Terminal": "144301",
"TrackID": "25119",
"payment_type": "KNET",
"service_type": "Payment Gateway",
"customerName": "User Name",
"customerEmail": "user@gmail.com",
"customerMobile": "98726012",
"customerCardType": null,
"customerCard": null,
"datetime": "2024-08-29 15:27:38"
}
]
}
HesabeRequestHandler
The payment data posted/passed via the checkout form is handled by HesabeRequestHandler. The posted data are first validated and encrypted in this file. The Encryption key and IV (Initialization Vector) taken from Profile page of Hesabe Merchant dashboard are used as the encryption keys.
The following sample PHP code snippets demonstrates how the process can be implemented in PHP Laravel.
Validation
$validator = $this->validate($request, [
'merchantCode' => 'required',
'access_code => 'required', // Only for Indirect Integration
'amount' => 'required|numeric|between:0.200,100000|regex:/^\d+(\.\d{1,3})?$/',
'paymentType' => 'required|in:0,1,2',
'responseUrl' => 'required|url',
'failureUrl' => 'required|url',
'version' => 'required'
]);
Loading Encryption Keys, Access Code, and API URLs from Config
$ivKey = HSB_IV_KEY;
$encryptionKey = HSB_ENCRYPTION_KEY;
$accessCode = HSB_ACCESS_CODE;
$checkoutApiUrl = HSB_CHECKOUT_API_URL;
$paymentUrl = HSB_PAYMENT_URL;
JSON Encoding
After Validation, the request data is JSON encoded which is the array of the request keys & values.
$requestDataJson = json_encode($request->input());
Encryption
This JSON data is then encrypted using AES algorithm with HesabeCrypto library
Invoking Hesabe Payment API “Checkout” endPoint
The accessCode is initialized in the Request header and the Request data is posted to the Hesabe Payment Gateway Checkout API endpoint
$baseUrl = http://sandbox.hesebe.com/api/
$checkoutApiUrl = {{$baseUrl}}/checkout
$checkoutRequestData = new Request([
'data' => $encryptedData
]);
$checkoutRequest = Request::create($checkoutApiUrl, 'POST', $checkoutRequestData->all());
$checkoutRequest->headers->set('accessCode', $accessCode);
Reading Response
$checkoutResponse = Route::dispatch($checkoutRequest);
$checkoutResponseContent = $checkoutResponse->content();
Decrytping Response & JSON Decoding
$decryptedResponse = HesabeCrypto::decrypt($checkoutResponseContent, $encryptionKey, $ivKey);
$responseDataJson = json_decode($decryptedResponse);